Ruby on Rails
In this guide, you'll learn how to add Rollout integrations in your Rails app.
Prerequisites#
To complete this guide, you will need:
- A Rollout account
- Docker: download from docker.com/get-started
- A local dev tunnel like ngrok
1. Create a Rollout project and run it locally#
Clone the starter template. You can clone it in the root of your Rails app or keep it in a separate repo:
# In your terminal$ git clone https://github.com/RolloutHQ/rollout-project-template.git rollout$ cd rollout# Install dependencies$ yarn
Tip: Remember to delete the git reference by calling
rm -rf .git
in the root of the Rollout project
To run your Rollout project locally:
- Run ngrok and forward to local port 3300
ngrok http 3300
- Open Docker Desktop
- Start running your Rollout project. In the root of your project, run the command:
You can find your project key at dashboard.rollout.com and your tunnel URL in step 1
$ yarn rollout dev --project-key=YOUR_PROJECT_KEY --tunnel-url=YOUR_TUNNEL_URL
When you go to your dashboard, you should see an environment labeled LOCAL
for your local development.
Clicking on this environment will let you see your local ROLLOUT_CLIENT_SECRET
:
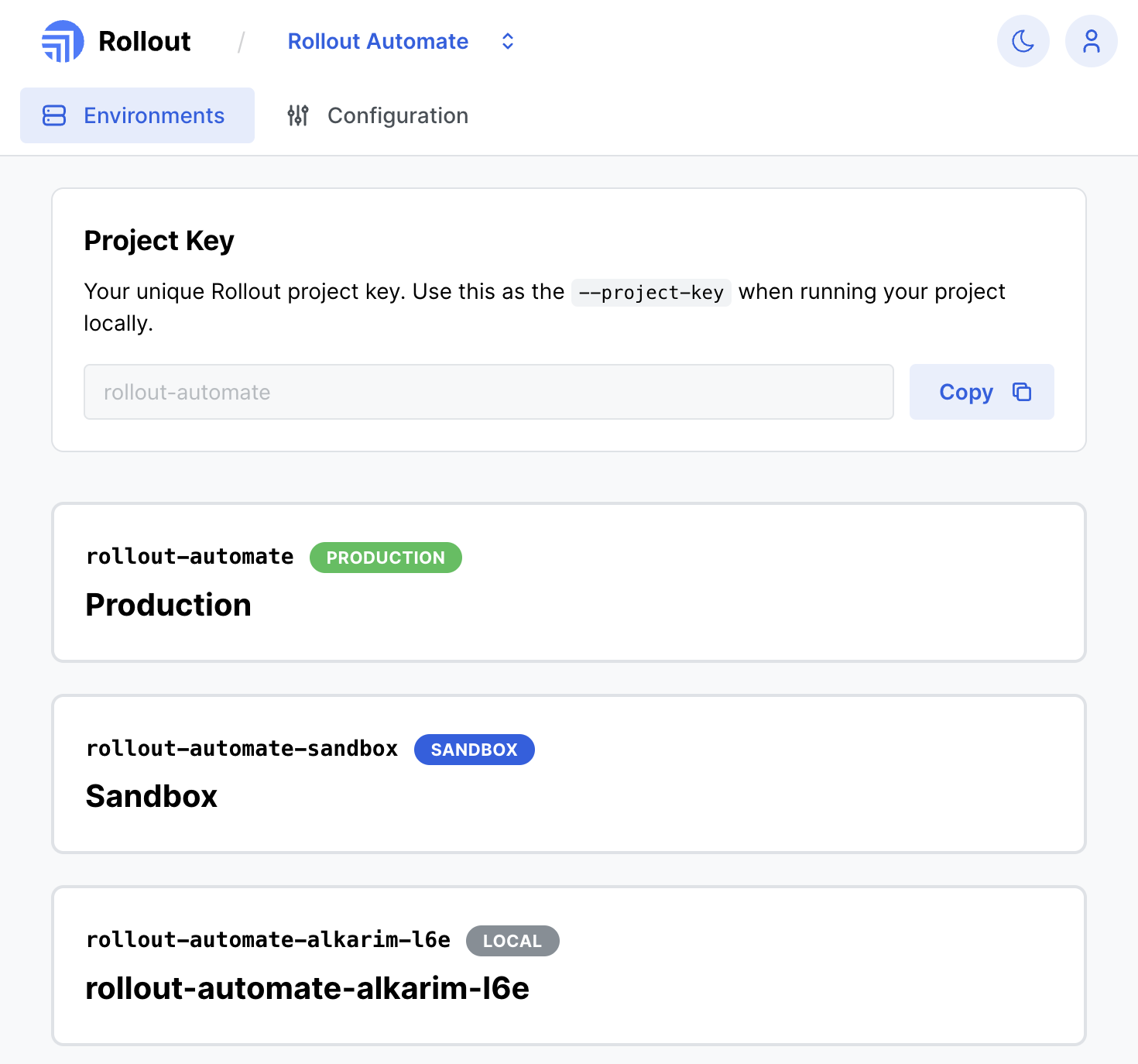
2. Create an Integrations page and generate a Rollout token#
You can render Rollout integrations on any page, for simplicity we will generate a new page and controller.
Add a new route
# In your config/routes/rbRails.application.routes.draw doget "/integrations", to: "integrations#index"end
Generate the controller
bin/rails generate controller Integrations index --skip-routes
Add the jwt package to your app
bundle add jwt
Finally, update your Integrations controller like so:
# In app/controllers/integrations_controller.rbrequire 'jwt'class IntegrationsController < ApplicationControllerdef indexgenerate_jwtenddef generate_jwt@rollout_token = JWT.encode({iss: ENV['ROLLOUT_PROJECT_KEY'], # Provided in the Rollout dashboardsub: current_user[:id].to_s, # Persistent ID for the current useriat: DateTime.now.to_i, # Time token was generatedexp: DateTime.now.to_i + 60 * 10000 # Token expiration},ENV['ROLLOUT_CLIENT_SECRET'], # Provided in the Rollout dashboard'HS512')endend
3. Render a Rollout UI Component in your app#
In your view, add the following code to render the AutomationsManager component
<div id="rollout-container"></div><script src="https://unpkg.com/@rollout/connect-react-dist@latest"></script><script>const container = document.getElementById("rollout-container");const apiBaseUrl = "http://localhost:3300/api";const token = "<%= @rollout_token %>";window.Rollout.defaultTheme();const ro = window.Rollout.init({token, apiBaseUrl });ro.renderAutomationsManager(container);</script>
Code Example#
Read the source code or try it yourself by running this example repository: github.com/RolloutHQ/rollout-rails-example