BlueprintEnabler
Description#
This component allows an end user to activate, deactivate and configure a specific blueprint.
Import#
import { BlueprintEnabler } from "@rollout/connect-react";
Usage#
Here's a basic usage example:
<BlueprintEnablerblueprintKey="BLUEPRINT_ID_TO_ACTIVATE"name="sample blueprint"prefilled={{ action: { appKey: "gmail" } }}/>
This will produce the following:
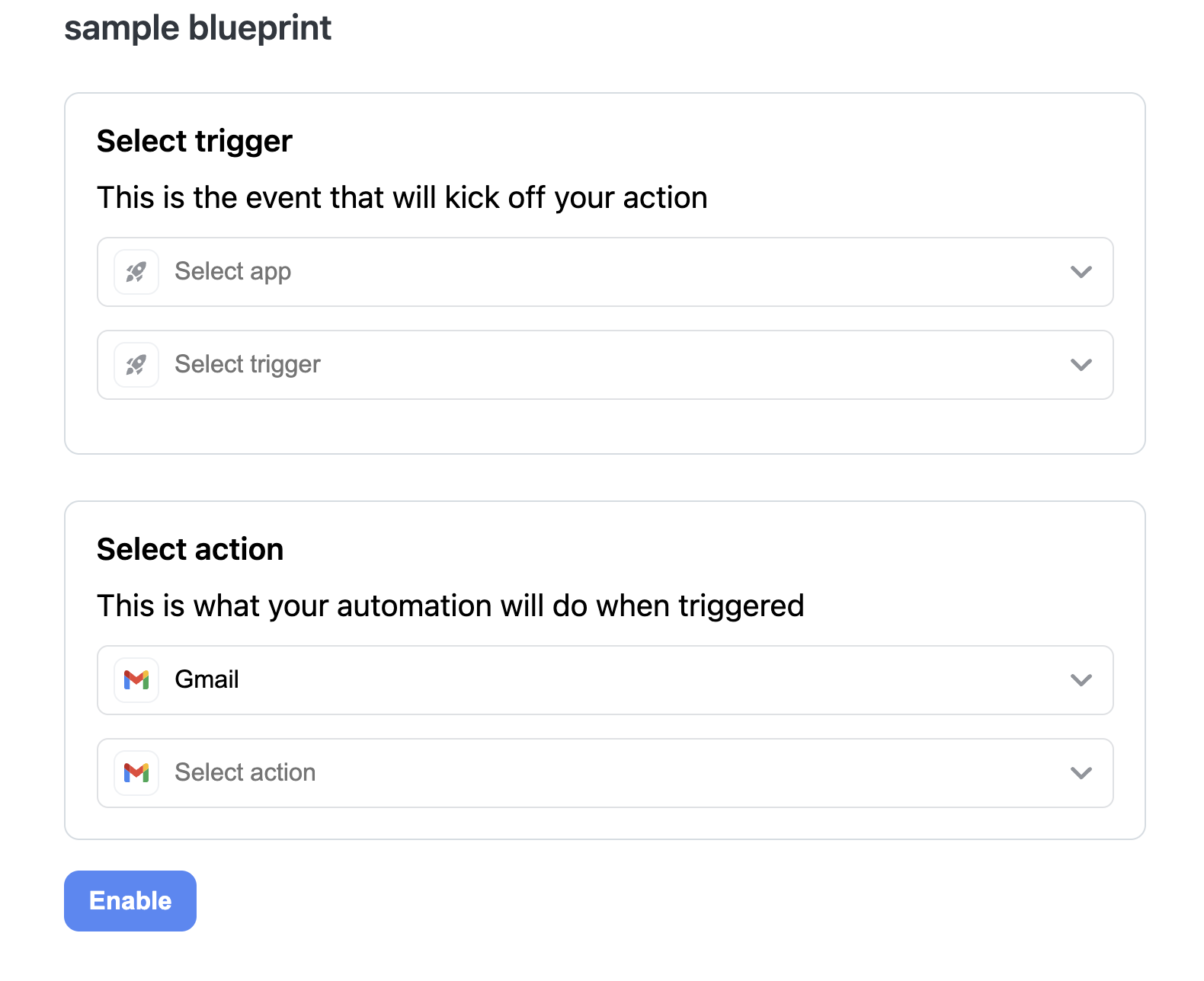
When the end users fills it in and presses the Enable button, it will look like this:
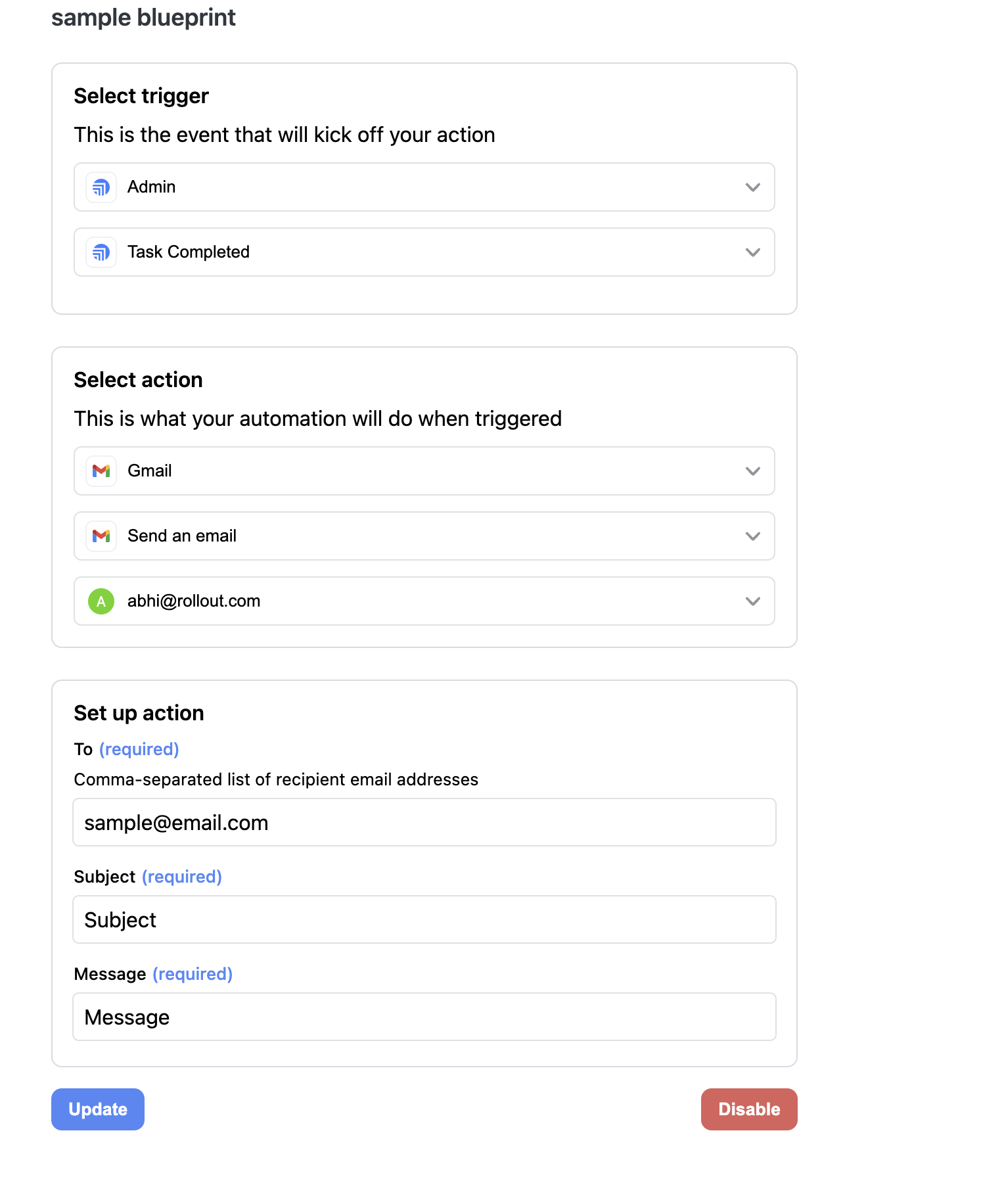
Pressing the Disable button will take it back to how it was before
We can also fill in more props. Here is a more complex example
<BlueprintEnablerblueprintKey="BLUEPRINT_ID_TO_ACTIVATE"name="complex sample blueprint"prefilled={{action: {appKey: "gmail",actionKey: "send-email",inputParams: {subject: "{{task_name}}",},},trigger: { appKey: "admin", triggerKey: "task-completed" },}}onEnabled={() => console.log("now enabled")}onDisabled={() => console.log("now disabled")}updateButtonText="Custom Update Button Text"enableButtonText="Custom Enable Button Text"disableButtonText="Custom Disable Button Text"/>
It will look like this when rendered:
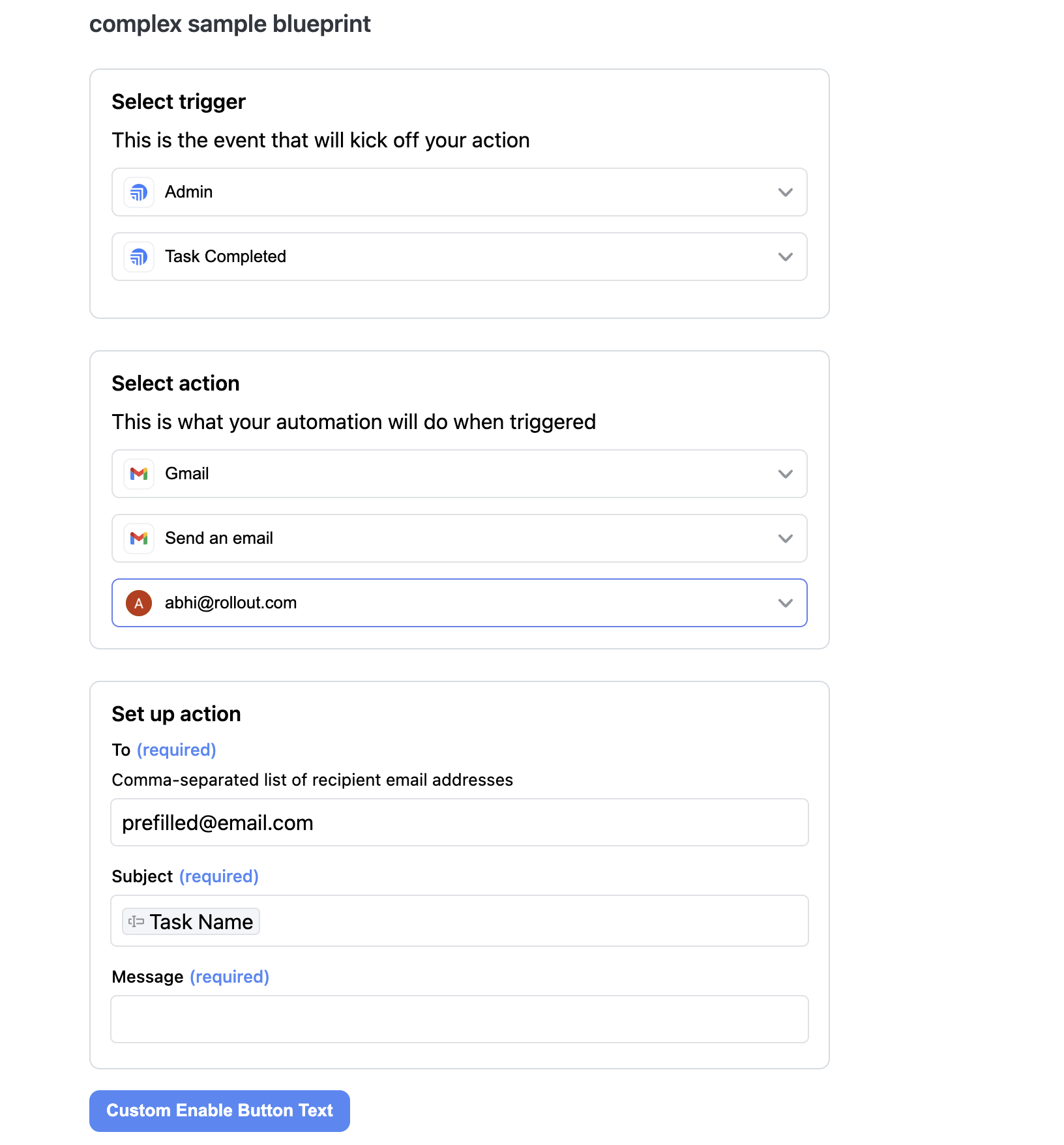
Props#
prop | type | required | Description |
---|---|---|---|
blueprintKey | string | true | A unique partner-defined identifier for the blueprint. (Obtainable via the dashboard by selecting the relevant Blueprint) |
name | string | true | The name of the blueprint to be displayed to the user |
prefilled | object | false | Data that can be prefilled for the blueprint automation. Please see the section on creating automations. |
getDefaultActionInputParams | function | false | See Dynamically Prefilling Actions. |
allowedActions | object | function | false | See Limiting Allowed Apps. |
allowedTriggers | object | function | false | See Limiting Allowed Apps. |
renderFields | object | false | See Customizing Automation Form. |
onUpdated | function | false | Callback function when the blueprint automation is updated |
onEnabled | function | false | Callback function when the blueprint automation is enabled |
onDisabled | function | false | Callback function when the blueprint automation is disabled |
onCancelUpdate | function | false | Callback when the user clicks the "Cancel" button while editing an enabled blueprint automation |
onCancelEnable | function | false | Callback function when the user clicks the "Cancel" button without enabling the blueprint automation |
enableButtonText | string | false | Button text for the enable button. Default 'Enable' |
disableButtonText | string | false | Button text for the disable button. Deafult 'Disable' |
updateButtonText | string | false | Button text for the renable button. Default 'Update' |