Handling HTTP Requests
It is often useful for Connectors to handle HTTP requests.
One common use case is populating a dropdown in the input UI of a Trigger or Action. Imagine that your app allows users to create forms and that your Rollout Connector supports a "Form Submitted" Trigger. You may want to enable users to select a specific form that will Trigger the automation. To do so, you'll need to fetch a list of forms in the user's account.
The http.ts
file is where you can handle requests like this.
Handling Requests#
An http.ts
file should export a function defined using the defineHTTPHandler
function from the Rollout Framework.
import { defineHTTPHandler, handleConsumerRequest } from "@rollout/framework";export const http = defineHTTPHandler(async (request) => {console.log("Handle request", request.url);const requestValidation = await handleConsumerRequest({token: request.headers.get("authorization")?.replace("Bearer ", "") ?? "",});if (!requestValidation.ok) {return Response.json(requestValidation.error, { status: 401 });}// return standard Response instancereturn Response.json(`Hello from http to ${requestValidation.consumer.consumerKey}`);});
The handler
function provided to defineHTTPHandler
should be a Fetch-compatible handler function.
It takes a standard Request instance and
returns an instance of Response.
Authentication#
The handleConsumerRequest
function can be used to validate a Consumer JWT and to find the relevant Consumer. In this example, the Consumer JWT is provided in the request's Authorization header.
Routing#
Your Request Handler will handle requests to <apiBaseUrl>/apps/<appKey>
. The API Base Url is specific to your Project Environment and can be found in the dashboard on the API tab.
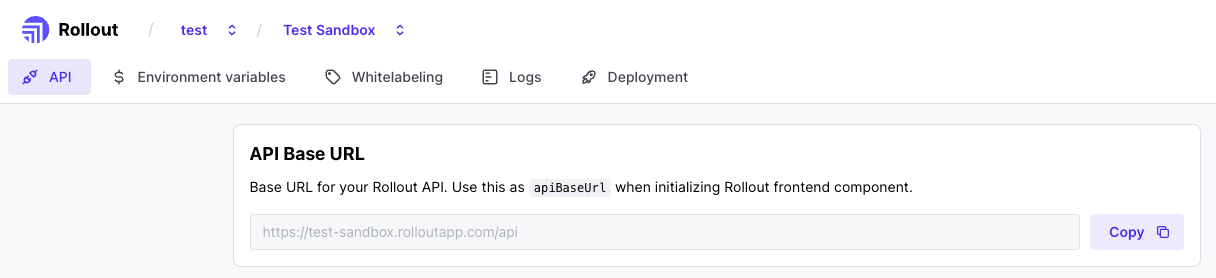
You can leverage fetch-compatible router libraries like itty-router and Hono to provide further routing within your Connector's HTTP Handler.
itty-router Example#
import { defineHTTPHandler } from "@rollout/framework";import { Router, json } from "itty-router";const router = Router().get("/hello-world", async () => json("Hello World!"));export const http = defineHTTPHandler(router.handle);
Hono Example#
import { defineHTTPHandler } from "@rollout/framework";import { Hono } from "hono";const router = new Hono().get("/hello-word", async (c) => c.json("Hello World!"));export const http = defineHTTPHandler(router.fetch);